참고 : Stanford CS193P : Lecture 03 https://youtu.be/w7a79cx3UaY
ViewController.swift
//
// ViewController.swift
// lecture1
//
// Created by Jho on 2020/03/20.
// Copyright © 2020 COMP420. All rights reserved.
//
import UIKit
class ViewController: UIViewController {
//var game = Concentration(numberOfPairsOfCards : (cardButtons.count +1_/2)//error
private lazy var game = Concentration(numberOfPairsOfCards: numberOfPairsOfCards)
var numberOfPairsOfCards: Int{ //IndexOfOneAndOnlyFaceUpCard가 계산되게(계산 프로퍼티)
get {
return (CardButtons.count+1)/2
}
}
private( set )var flipCount=0{
didSet {
flipCountLabel.text = "Flips : \(flipCount)"
}
}
@IBOutlet private weak var flipCountLabel: UILabel!
@IBOutlet private var CardButtons: [UIButton]!
@IBAction private func touchcard(_ sender: UIButton) {
flipCount+=1; //property oberver to observe flipCount property
if let cardNumber = CardButtons.firstIndex(of: sender){
game.chooseCard(at: cardNumber)
updateViewFromModel()
}else{
print("Chosen Card was not in cardButtons")
}
}
private func updateViewFromModel(){
for index in CardButtons.indices {
let button = CardButtons[index]
let card = game.cards[index]
if card.isFaceUP{
button.setTitle(emoji( for: card),for: UIControl.State.normal)
button.backgroundColor = #colorLiteral(red: 1.0, green: 1.0, blue: 1.0, alpha: 1.0)
}
else {
button.setTitle("", for: UIControl.State.normal)
button.backgroundColor=card.isMatched ? #colorLiteral(red: 1, green: 0.5763723254, blue: 0, alpha: 0) : #colorLiteral(red: 1, green: 0.5763723254, blue: 0, alpha: 1)
}
}
}
private var emojiChoices: Array<String> = [ "🦇", "😱", "🙀", "😈", "👻", "🎃", "🍭", "🍬", "🍎" ]
private var emoji = [Int:String]() //dictionary
private func emoji(for card : Card) -> String {
if emoji[card.identifier] == nil,emojiChoices.count > 0{ //Optional type -> nil처리를 해줘야함
emoji[card.identifier]=emojiChoices.remove(at: emojiChoices.count.arc4random)
}
return emoji[card.identifier] ?? "?"
}
}
extension Int {
var arc4random: Int {
if self > 0 {
return Int(arc4random_uniform(UInt32(self))) // self is Int
} else if self < 0 {
return -Int(arc4random_uniform(UInt32(abs(self))))
}
else {
return 0
}
}
}
Concentraion.swift
//
// Concentration.swift
// lecture1
//
// Created by Jho on 2020/03/27.
// Copyright © 2020 COMP420. All rights reserved.
//
import Foundation
class Concentration
{
// 클래스의 프로퍼티가 초기값을 가지는 인자없는 free initializer를 가짐 초기화 충분하지 않음
private ( set ) var cards = [Card]()
private var indexOfOneAndOn1yFaceUPCard : Int? {
get {
var foundIndex: Int?
for index in cards.indices {
if cards[index].isFaceUP {
if foundIndex == nil {
foundIndex = index
} else {
return nil
}
}
}
return foundIndex
}
set { //(newValue) 생략가능
// true for the touched card. false for the other cards
for index in cards.indices {
cards[index].isFaceUP = (index == newValue)
}
}
}
//var cards : Array<Card> = []
// var cards = Array<Card>() //empty
// var indexOfOneAndOnlyFaceUpCard : Int?
func chooseCard(at index:Int){
assert(cards.indices.contains(index),"Concentration.chooseCard(at: \(index)): chosen index not in the cards")
if !cards[index].isMatched{
//check if card match
if let matchIndex = indexOfOneAndOn1yFaceUPCard, matchIndex != index{ //check if card match
if cards[matchIndex].identifier == cards[index].identifier{
cards[matchIndex].isMatched = true
cards[index].isMatched = true
}
cards[index].isFaceUP = true
} else{
// either no cards or 2 cars are faced up
indexOfOneAndOn1yFaceUPCard = index
}
//cards[index].isFaceUp = !cards[index].isFaceUp
}
}
init(numberOfPairsOfCards : Int) {
assert(numberOfPairsOfCards > 0, "Concentration.init(at: \(numberOfPairsOfCards)): you must have at least one pair of cards")
for _ in 1...numberOfPairsOfCards {
let card = Card()
cards += [card, card]
// cards.append(card)
// cards.append(card)
}
//TODO : Shuffle the cards
for _ in 1...cards.count {
let randomIndex = Int (arc4random_uniform(UInt32(cards.count)))
cards.swapAt(0, randomIndex)
}
}
}
Card.swift
//
// Card.swift
// lecture1
//
// Created by Jho on 2020/03/27.
// Copyright © 2020 COMP420. All rights reserved.
//
import Foundation
struct Card
{
var isFaceUP = false
var isMatched = false
var identifier: Int
private static var identifierFactory = 0
private static func getUniqueIdentifier() -> Int {
identifierFactory += 1
return identifierFactory
}
init(){
self.identifier = Card.getUniqueIdentifier()
}
}
실행결과
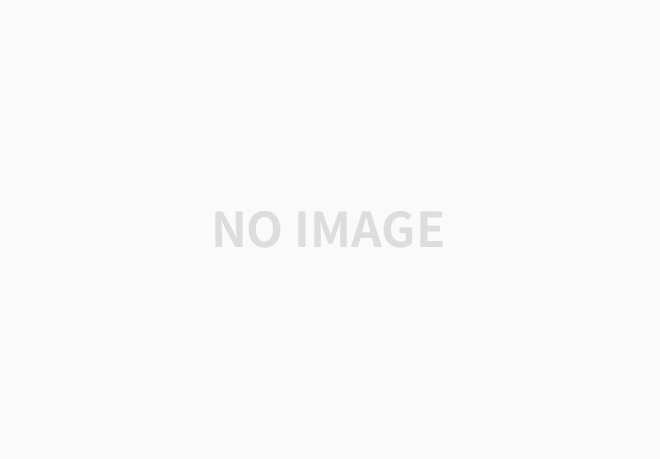